Intro to Dart Data Types
Data types are the building blocks of every programming language. In Dart, a data type is a variable that holds a value. It's very similar to the variables in math.
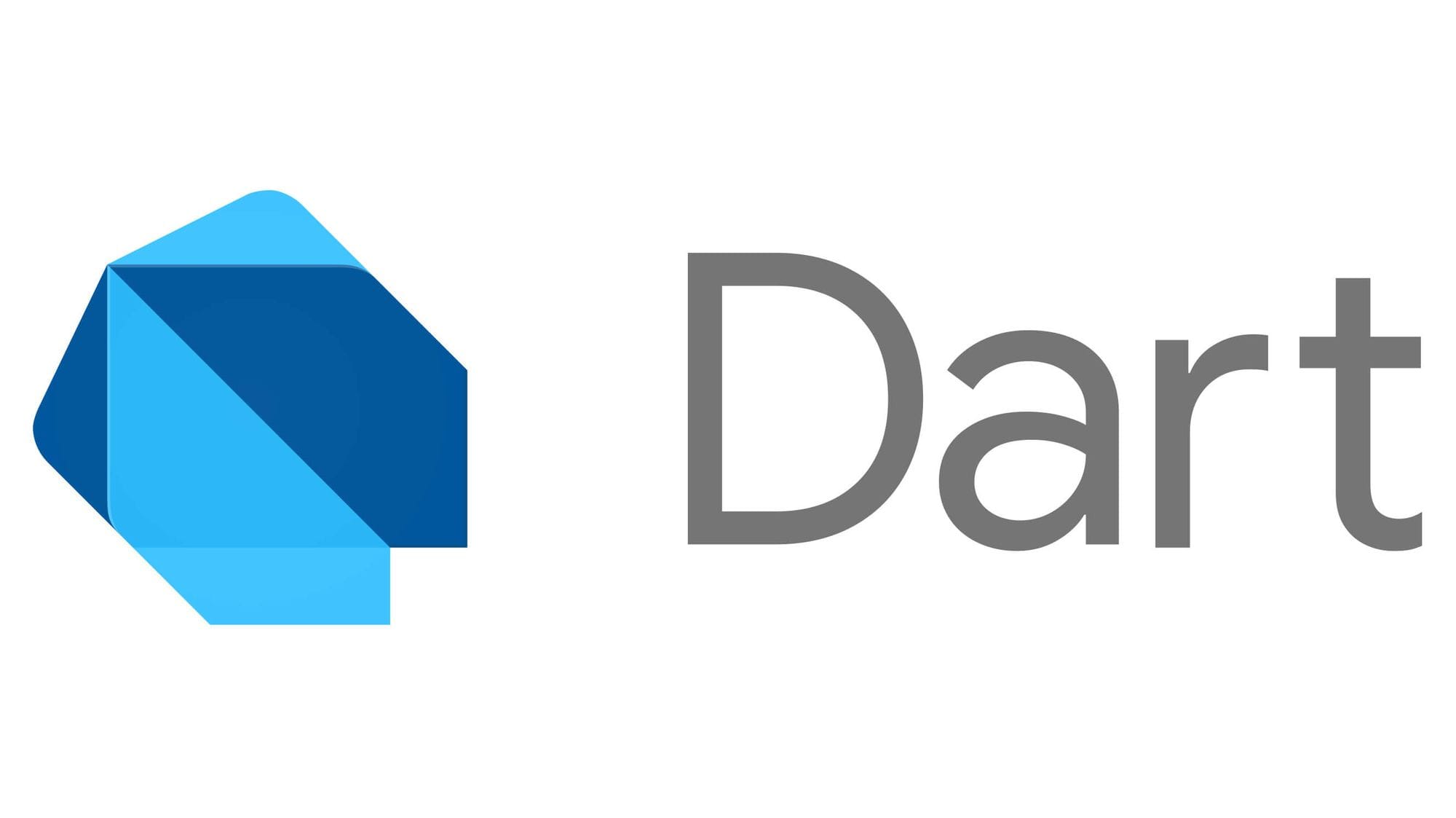
Data types are the building blocks of every programming language. In Dart, a data type is a variable that holds a value. It's very similar to the variables in math. Think about the x
variable in math. x
can be a representation of a certain value - a number. In Computer Science, that certain value can represent a lot more things than a number; the value can be a text, the value can be either true
or false
, or it can represent other values. Let's take a look at the syntax of declaring variables in dart:
(Data Type) (Variable Name) = Value;
int age = 100;
^ ^ ^
| | |
(Data Type) (Variable Name) = Value;
Examples:
bool isSmart = true;
String message = "Hello World";
double pi = 3.14;
Let's take a look at the most commonly used data types in Dart. Try them out in DartPad.
Boolean
A boolean is a data type that can hold either one of the following values - true
or false
. It's usually used in conditional statements. The boolean type is denoted by the shortcut bool
.
Example usage:
void main() {
bool isRaining = true;
if (isRaining) {
print("It's currently raining.");
} else {
print("It's not raining right now.");
}
}
String
A string is a data type that represents a bunch of text. In dart, it holds a sequence of UTF-16 code units. You can use single or double quotes to create a string:
void main() {
String message = "Hello World";
print(message);
}
Integer
An integer is a data type that represents a whole number. In dart, the integer is 64-bit. The integer type is denoted by the shortcut int
.
Example usage:
void main() {
int x = 4;
int y = 3;
print(x + y);
}
Double
A double is a data type that can represent numbers with decimals.
Example usage:
void main() {
double x = 3.85;
double y = 2.18;
print(x + y);
}
Lists
A list is a data type that can represent a list of variables. The list type is denoted by the shortcut List<T>
, where T
is a data type. If I want to represent a list of integers, the list type should be denoted as List<int>
.
Example usage:
void main() {
List<int> numbers = [1, 3, 5, 7, 9, 11];
int total = 0;
for (int number in numbers) {
total+=number;
// total+=number; is a shortcut for total = total + number;
}
print(total);
}
It is also possible to access the element of a list using list[index]
, where index is the (n-1)th
element of the list. For example, if I want to access the 1st element of the list, I would do so using list[0]
, and if I want to access the 2nd element of the list, I would do so using list[1]
. Note that index
starts at 0 instead of 1.
Example usage:
void main() {
List<int> numbers = [1, 3, 5];
print(numbers[0]); // Output : 1
print(numbers[1]); // Output : 3
print(numbers[2]); // Output : 5
}
Map
A map is a data type that holds key-value pairs.
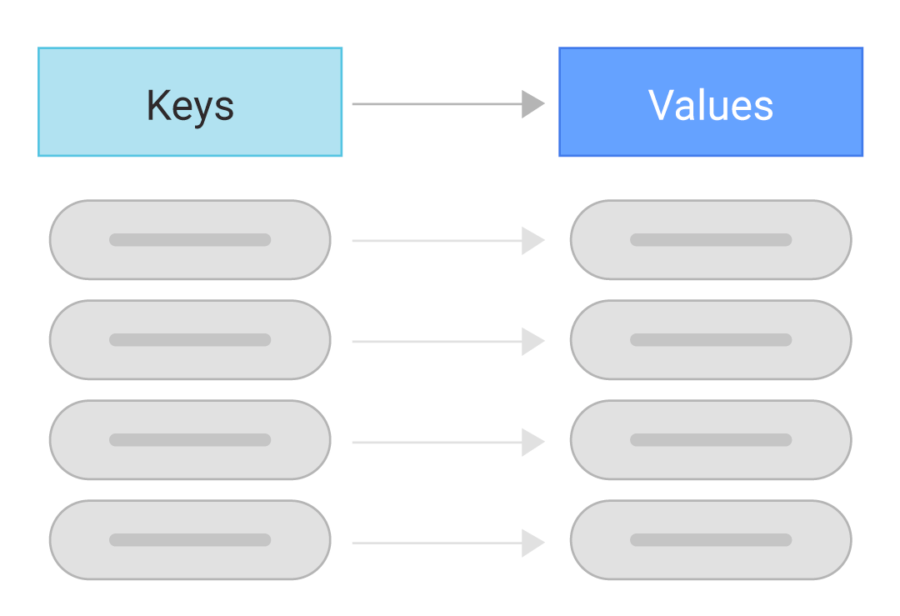
Maps allows you to quickly lookup a certain value by referencing its key. The syntax is as follows: map["key"]
For example:
void main() {
Map<String, String> user = {
"firstName": "Bill",
"lastName": "Gates",
"username": "BGates",
};
print(user["firstName"]); // Output: Bill
print(user["lastName"]); // Output: Gates
print(user["username"]); // Output: BGates
}
Sources:
- Dart programming language. (n.d.). Retrieved from https://dart.dev/
- The Dart Type System. (n.d.). Retrieved from https://dart.dev/guides/language/type-system
- Int class. dart. (n.d.). Retrieved from https://api.dart.dev/stable/2.6.1/dart-core/int-class.html
- Alastairn. (2023, August 15). What is a Key Value Database? Definition & FAQs | ScyllaDB. ScyllaDB. https://www.scylladb.com/glossary/key-value-database/